23 March, 2025
Minimal POC: Next.js Middleware Vulnerability
A minimal proof-of-concept demonstrating a vulnerability in Next.js middleware described in CVE-2025-29927
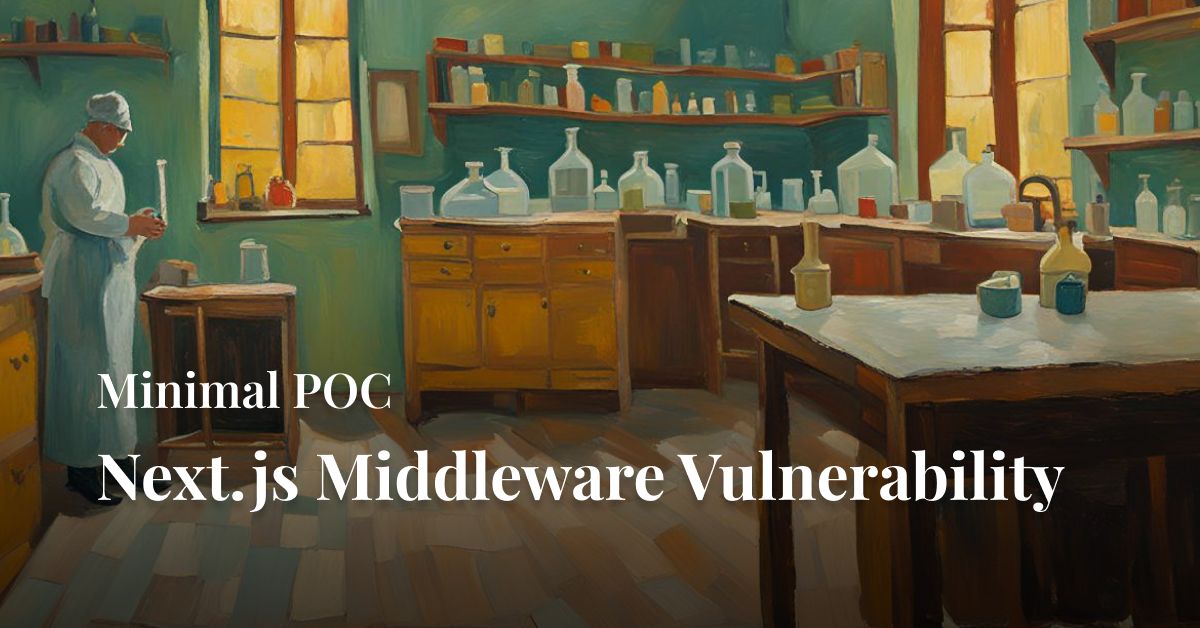
- Vulnerability Description
- Proof-of-Concept
- Who Might Be Affected
- How It Works
- The Fix
- Conclusion
During the weekend, I came across a tweet from a security researcher regarding a vulnerability in Next.js middleware. There was a lot of discussion about it, but often without a deep understanding of the issue. Therefore, I decided to create a minimal proof-of-concept to clearly demonstrate the vulnerability.
The aim is to show that while the vulnerability is indeed critical, its real-world impact might not be as significant as some believe.
IMPORTANT: I am not affiliated with the Next.js team. This article is strictly for educational purposes.
Vulnerability Description
CVE-2025-29927: A critical vulnerability exists in Next.js middleware that allows attackers to bypass authorization checks by manipulating the x-middleware-subrequest
header. This flaw affects Next.js versions prior to 14.2.25 and 15.2.3, potentially granting unauthorized access to sensitive resources.
Links:
Proof-of-Concept
To demonstrate this vulnerability, I built a simple Next.js application with middleware that is designed to always block user access to the main page (/
). The middleware is defined in middleware.ts
:
import { NextResponse } from 'next/server'
import type { NextRequest } from 'next/server'
export function middleware(request: NextRequest) {
return NextResponse.rewrite(new URL('/rejected', request.url))
}
export const config = {
matcher: '/:path*',
}
Next, create a new page at app/rejected/page.tsx
:
export default function Rejected() {
return (
<div>
No you are not allowed access to this page
</div>
);
}
And add a short message to the main page at app/page.tsx
:
export default function Home() {
return (
<div>
OK you get it
</div>
);
}
Now, run the server using npm run dev
and try to access the main page (/
). You should see:
However, by simply adding the x-middleware-subrequest
header with the value middleware:middleware:middleware:middleware:middleware
, you can bypass the middleware and successfully access the main page:
Who Might Be Affected
Theoretically, this vulnerability could affect codebases that rely on Next.js middleware for access control. For instance, middleware can be used in scenarios like these to redirect to a login page or prevent access to specific routes:
- Controlling access to assets (images, PDFs, etc.) based on user authentication.
- Redirecting unauthenticated users to a login page.
- Restricting access to admin routes for non-admin users.
However, in practice, pages further down the request chain might depend on data provided by the middleware for authorization decisions, such as User ID or Role. If the middleware is bypassed, these pages might simply crash due to missing expected data, effectively preventing unauthorized access anyway.
Furthermore, websites using Next.js for static content generation (SSG) - like this blog - are not affected by this vulnerability.
How It Works
By examining the Next.js source code, we can pinpoint the location where the x-middleware-subrequest
header is processed:
Source code: next/src/server/web/sandbox/sandbox.ts#L94
export const run = withTaggedErrors(async function runWithTaggedErrors(params) {
const runtime = await getRuntimeContext(params)
const subreq = params.request.headers[`x-middleware-subrequest`]
const subrequests = typeof subreq === 'string' ? subreq.split(':') : []
const MAX_RECURSION_DEPTH = 5
const depth = subrequests.reduce(
(acc, curr) => (curr === params.name ? acc + 1 : acc),
0
)
if (depth >= MAX_RECURSION_DEPTH) {
return {
waitUntil: Promise.resolve(),
response: new runtime.context.Response(null, {
headers: {
'x-middleware-next': '1',
},
}),
}
}
...
The crucial part is the depth
variable. It's calculated by counting how many times the current middleware's name appears in the x-middleware-subrequest
header. If this depth
reaches or exceeds MAX_RECURSION_DEPTH
, the middleware is bypassed.
The Fix
The vulnerability has been addressed in Next.js versions 14.2.25 and 15.2.3 by:
- Filtering out this header from incoming requests: next/src/server/lib/server-ipc/utils.ts#L53
- Introducing a new header,
x-middleware-subrequest-id
, containing a random value. This prevents users from tampering with the header: next/src/server/lib/router-server.ts#L170
Conclusion
While this vulnerability in Next.js middleware is indeed critical, its real-world impact might be less significant than initially perceived by some. The fix is straightforward and can be easily implemented in existing codebases by upgrading Next.js. However, for robust authentication and authorization in Next.js applications, it remains best practice to utilize dedicated solutions like NextAuth.js.